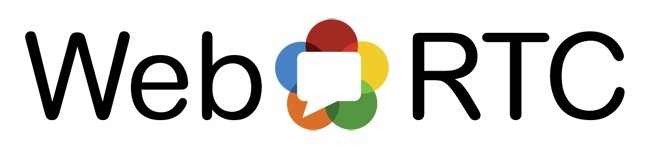
Your typical applications:
Think outside the box:
<video id="sourcevid" autoplay muted></video>
var video = document.getElementById('sourcevid'); var constraints = { "video": true, "audio": true }; navigator.getUserMedia(constraints, success, error); function success(stream) { video.src = window.URL.createObjectURL(stream); }
Requirements: a Web page, a USB camera, and
a Web server = Apache
Shout-out to the Team:
<script src="//path/to/rtc.js"></script> <div id="videos"></div>
var rtcOpts = { room: 'wdcnz', signaller: '//switchboard.rtc.io' }; var rtc = RTC(rtcOpts); var videos = document.getElementById('videos'); videos.appendChild(rtc.local); videos.appendChild(rtc.remote);
Mesh topology, unless you use an MCU
Open Source MCU: Janus
Requirements: a Web page, a Web server, a USB camera on both ends
Additionally: a signalling server = rtc-switchboard
Payload: SDP packet (Session Description Protocol)
Options for a Signalling Server:
...based on Primus.js on node.js
Primus is a universal wrapper for real-time frameworks
Setup:
git clone https://github.com/rtc-io/rtc-switchboard.git
cd rtc-switchboard
npm install
SERVER_PORT=8997 node server.js
...and use it in our example
Browsers have built-in diagnostics for RTCPeerConnection:
Understand your SDP packets:
http://webrtchacks.com/sdp-anatomy/
Check your Internet Connection:
http://netscan.co/
Use rtc-quickconnect module for your development
Example multi-peer application:
rtcio-demo-quickconnect
Requirements: a Web page, a Web server, a USB camera on both ends, a signalling server
Additionally: a STUN server (Session Traversal Utilities for NAT)
Requirements: a Web page, a Web server, a USB camera on both ends, a Signalling Server
Additionally: a STUN/TURN server (Traversal Using Relay NAT)
Add to the setup of the RTC object:
var rtcOpts = {
room: 'wdcnz',
signaller: '//switchboard.rtc.io',
iceServers: [
{ url: 'stun:rtcjs.io' },
{ url: 'stun:stun.l.google.com:19302'},
{ url: 'turn:turn.server:3478', credential: 'passwd', username: 'user'}
]
};
var rtc = RTC(rtcOpts);
with Google STUN and TURN servers
Using a browser on the phone
RTCDataChannel API similar to WebSocket API
function bindDataChannelEvents(id, channel, attributes, connection) {
channel.onmessage = function (evt) {
messages.innerHTML = evt.data;
};
messages.onkeyup = function () {
channel.send(this.innerHTML);
};
}
rtc.on('ready', function(session) {
session.createDataChannel('chat');
session.on('channel:opened:chat', bindDataChannelEvents);
});
For pure DataChannel use, remove audio/video capture:
<script src="path/to/rtc.min.js"></script> <div id="messages" contenteditable></div>
var rtcOpts = { room: 'wdcnz-data', signaller: '//switchboard.rtc.io', capture: false }; var rtc = RTC(rtcOpts);
Telehealth application with shared whiteboard and shared pointers
Project by Feross Aboukhadijeh (PeerCDN - sold to Yahoo)
Develop a BitTorrent client for the Web using RTCDataChannel